Geotab Map Add-In Overview
Map Add-ins are integrations embedded within the Map or Trips History pages of the MyGeotab platform. Once installed, a panel will appear on the right-side of the Map or Trips History page, containing the UI of the Map Add-in. These Add-ins can read from and operate on the map, as well as access the MyGeotab APIs. This document outlines the installation process and APIs available for Map Add-In development.
Below is an example of a Map Add-In installed within the Map page of MyGeotab. When a user clicks a vehicle on the map, this integration displays the relevant data for that vehicle.
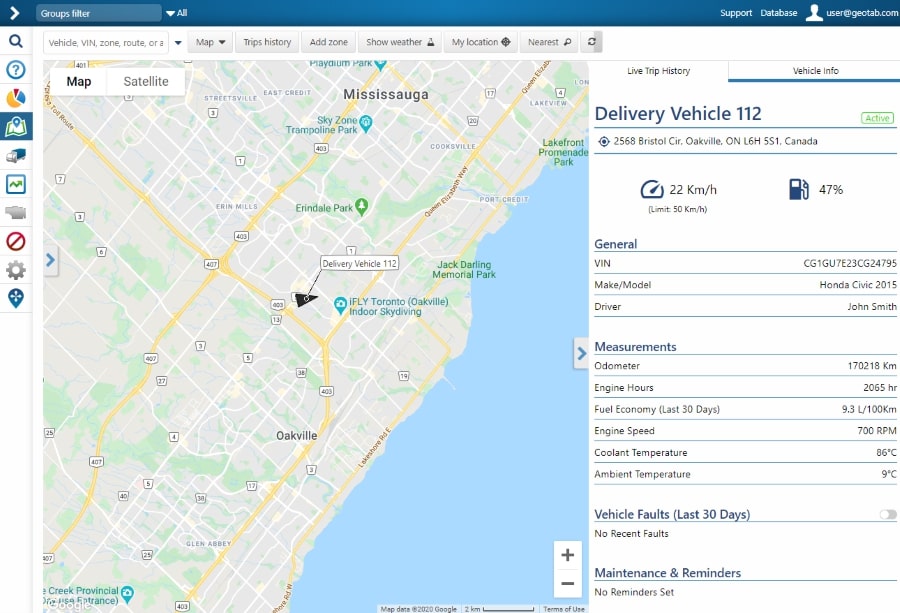
Installation
Map Add-ins are installed by uploading an Add-in Configuration file into MyGeotab. Click on Administration -> System... -> System Settings -> Add-Ins -> New Add-In, and upload the Add-in Configuration file. The following is an example of a Map Add-in's Configuration file:
{
"name": "Tooltip",
"supportEmail": "yourSupportEmail@company.com",
"version": "1.0",
"items": [{
"page": "map",
"title": "Tooltips",
"noView": false,
"mapScript": {
"src": "addin.js",
"style": "addin.css",
"url": "addin.html"
}
}]
}
The main properties of the Configuration file are as follows:
- page - This defines which page the Map Add-in will reside within. This could be “map” (The Map page) or “tripsHistory” (the Trips History page). The default value is “map”.
- title - This is a heading displayed at the top of the panel when there are multiple Map Add-ins installed. If a "title" is not provided, the Add-in defaults to the "name" parameter.
- noView - If true, the add-in will not be displayed in the right-side panel. The default value is false.
- src - The JavaScript file reference for the Add-in. This can be an externally hosted file or uploaded into MyGeotab by dragging and dropping it into the Configuration file window.
- style - The CSS file reference for this Add-In. This can be externally hosted or uploaded to MyGeotab.
- url - The HTML file reference for this Add-In. This option can be used instead of src and style. Links to CSS and JavaScript files can be made within this HTML file. All content within the <body> tags will be added to the Map Add-in UI ((Example).
You can find example Map Add-ins here.
Usage
Iframe Setup
In the MyGeotab portal, Map Add-ins are loaded when the user visits the MyGeotab page containing the Add-in. For example, the Add-in in Figure #1 loads when the user visits the Map page.
Map Add-ins are loaded inside an iframe with its sandbox attribute set to "allow-scripts". This allows the Map Add-in to run custom scripts, such as the JavaScript file from the "src" parameter in the Add-in Configuration file.
Important Notes:
- All Map Add-ins installed in a database are active at the same time, when the user is on the Map or Trips History page. If different Add-ins use the same variable name, it will cause an error and will lead to a non-working Add-in. You should name your variables according to your solution name - e.g. for "ABC Add-in" you should use "abc" as a prefix for all your variables.
- When the user leaves the page, the content of the iframe is cleared by the browser.
- When the user returns to the page, the scripts inside the iframe are reloaded.
- If necessary, the current Add-In state can be saved in Local Storage, IndexedDB, or elsewhere.
Do not access elements or APIs outside the iframe panel; they can be changed without notice in future versions of MyGeotab, which might break your Add-In. Use only the services and methods documented here and on the API Reference page when interacting with a page outside the iframe.
Panel Size
The iframe panel has a fixed width of 450 pixels; the width shrinks to accomodate screens with widths below 600 pixels. The panel height is responsive, always reaching the bottom of the page.
The figures below display how the panel size changes between a desktop screen and a mobile phone screen.
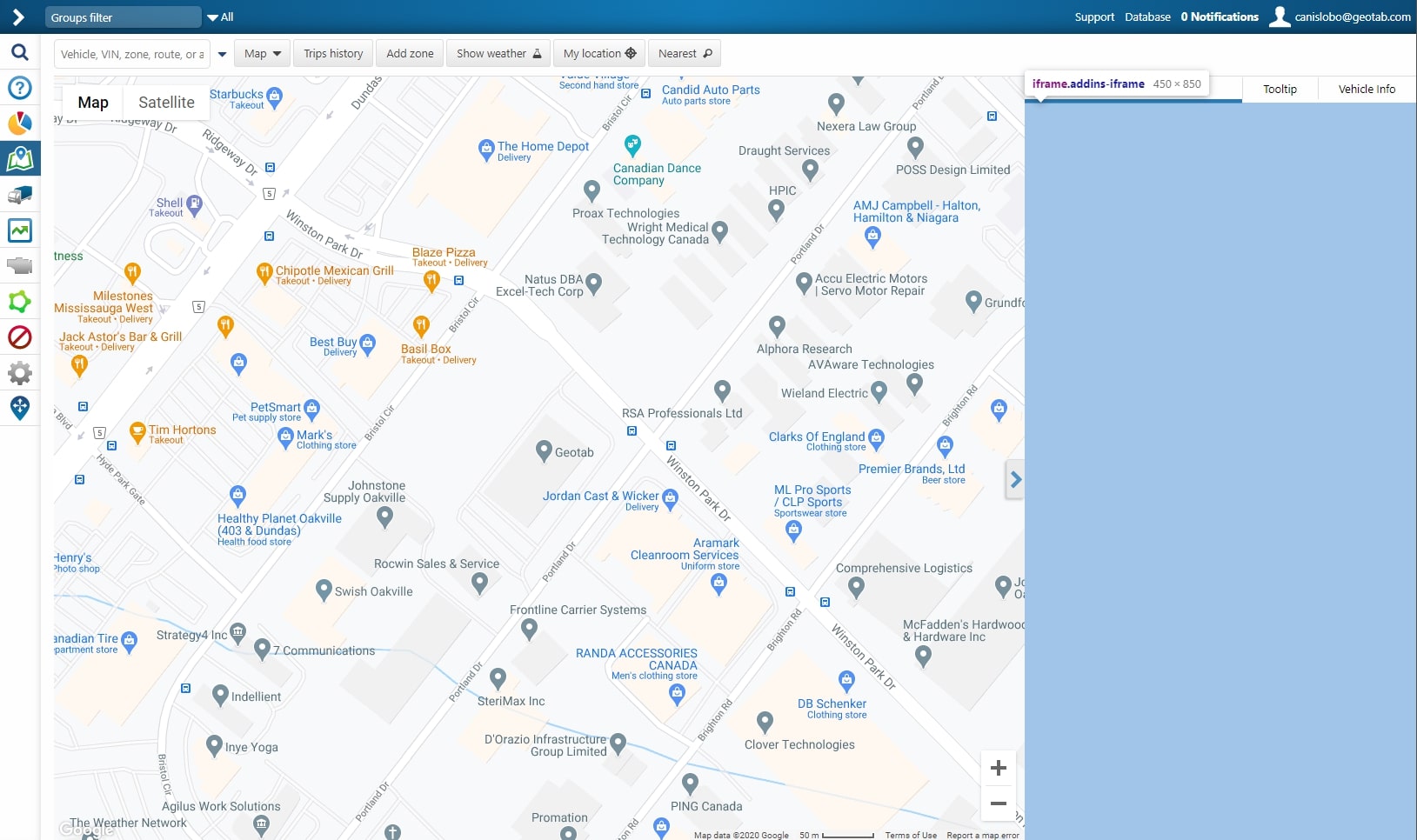
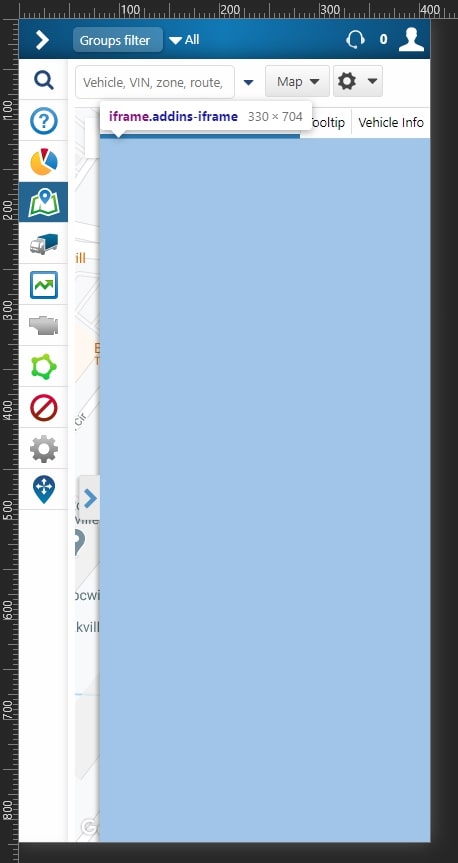
If multiple Map Add-ins are installed, each is accessible by selecting the appropriate tab at the top of the panel. In Figure #4, there are 3 add-ins installed on the page, denoted by the 3 tabs at the top of the panel. The add-ins are titled "Live Trip History", "Tooltip", and "Vehicle Info".
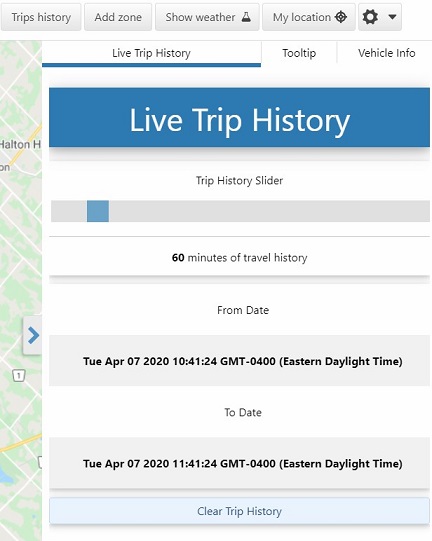
Structure
The starting point for the Map Add-in JavaScript code is the function that is added as a method to "window.geotab.addin". For example:
geotab.addin.request = (elt, service) => {
// code for Map Add-In
}
This function has two arguments:
- elt - The HTMLElement of the Add-In. It contains everything that belongs to the Add-In.
- service - The JavaScript file reference for the Add-in.
The Add-in function will be called when a user visits the Add-in by clicking on its tab. After that, the "focus" and "blur" events will be fired when the user opens or leaves the Add-in tab, respectively (See Page Service).
Map Add-In Services
page service (Example)
Service for getting/setting page state and moving between MyGeotab page
Methods
Service methods | Description |
---|---|
set (key: string, value: string): Promise<boolean> | Sets new key/value pair to page state |
get (): Promise<object> | Gets page state |
go (page: string, state?: object): Promise<boolean> | Changes current page |
hasAccessToPage (page: string): Promise<boolean> | Checks whether current user has access to MyGeotab page |
getFilterState (): Promise<IGroupFilterId[]> | Gets current company filter state |
attach (eventName: string, eventHandler: (serviceData: any) => void): void | Attaches event handler to service |
detach (eventName: string, eventHandler?: (serviceData: any) => void): void | Detaches event handler to service |
Properties
Service property name | Type | Description |
---|---|---|
active | boolean | Current map add-in state. It's true if current add-in is focused |
Events
Event name | Event object | Description |
---|---|---|
focus | Empty object | Event is fired when user focuses on current map add-in |
blur | Empty object | Event is fired when user focuses on another map add-in |
stateChange | state: (object) - Updated page state | Event is fired when the state of the page is changed |
filterChange | groups: (IGroupFilterId[]) - Updated array of filter groups | Event is fired when the company filter groups is changed |
Interface IGroupFilterId
Group id object that is selected by user in company filter
Property | Type |
---|---|
id | string |
localStorage service (Example)
Service to request information stored in browser LocalStorage
Methods
Service methods | Description |
---|---|
set (key: string, value: string): Promise<boolean> | Sets key-value pairs to browser localStorage with key |
remove (key: string): Promise<boolean> | Removes value from browser localStorage by key |
get (key: string): Promise<string> | Gets value from browser localStorage by key |
api service (Example)
Service for requesting data from Geotab server
Methods
Service methods | Description |
---|---|
call (method: string, params: object): Promise<any[]> | Sends single request to Geotab server |
multiCall (calls: TApiCall[]): Promise<any[][]> | Sends multiple requests to Geotab server in one batch |
getSession (): Promise<ISessionInfo> | Gets current user session information |
Interface ISessionInfo
Current user session information
Property | Type |
---|---|
database | string |
userName | string |
sessionId | string |
domain | string |
Type TApiCall
[string, object]
Tuple for calling server methods where first element is method name and second is an object with parameters.
events service (Example)
Service for catching events that happens when user interact with different entities on the map
Methods
Service methods | Description |
---|---|
attach (eventName: string, eventHandler: (serviceData: any) => void): void | Attaches event handler to service |
detach (eventName: string, eventHandler?: (serviceData: any) => void): void | Detaches event handler to service |
Events
Event name | Event object | Description |
---|---|---|
move | data: (ICoordinate) - Position of the pointer on the map | Event is fired when user moves pointer over the map |
over | data: (TEventData) - Main information about entity | Event is fired when user moves pointer over an object on the map |
out | data: (TEventData) - Main information about entity | Event is fired when user moves pointer out of an object on the map |
click | data: (TEventData) - Main information about entity | Event is fired when user clicks on an object on the map |
change | data: (TEntityEventData) - Main information about entity and its state | Event is fired when status of the entity on the map is changed |
Interface IZoneEvent
Event object that is sent to add-in when user interacts with zone
Property | Type |
---|---|
type | "zone" |
entity | IZoneEventData |
Interface IZoneEventData
Zone object that is sent to add-in when user interacts with zone
Property | Type |
---|---|
id | string |
Interface IDeviceEvent
Event object that is sent to add-in when user interacts with device
Property | Type |
---|---|
type | "device" |
entity | IDeviceEventData |
Interface IDeviceEventData
Device object that is sent to add-in when user interacts with device
Property | Type |
---|---|
id | string |
Interface IRouteEvent
Event object that is sent to add-in when user interacts with route
Property | Type |
---|---|
type | "route" |
entity | IRouteEventData |
Interface IRouteEventData
Route object that is sent to add-in when user interacts with route
Property | Type |
---|---|
id | string |
Interface ITripEvent
Event object that is sent to add-in when user interacts with trip
Property | Type |
---|---|
type | "trip" |
entity | ITripEventData |
Interface ITripEventData
Trip object that is sent to add-in when user interacts with trip
Property | Type | Description |
---|---|---|
id | string | Id of the trip |
device | { id: string; } | Device id object that drove this trip |
dateTime | string | Date and time of the trip point |
Interface IExceptionsEvent
Event object that is sent to add-in when user interacts with device exceptions
Property | Type |
---|---|
type | "exceptions" |
entity | IExceptionsEventData |
Interface IExceptionsEventData
Exceptions object that is sent to add-in when user interacts with exception icon on the map
Property | Type |
---|---|
exceptions | IExceptionEventData[] |
Interface IExceptionEventData
Exception object that is sent to add-in when user interacts with exception icon on the map
Property | Type | Description |
---|---|---|
to | string | Date and time when this exception ends |
from | string | Date and time when this exception starts |
id | string | Id of the exception |
rule | { id: string; } | Rule id object of this exception |
device | { id: string; } | Device id object where this exception happen |
Interface IDeviceChangeEvent
Event object that is sent to add-in when vehicle location on the map changes
Property | Type |
---|---|
type | "device" |
entity | IDeviceEventData |
visible | boolean |
location | ILocation |
Type TEventData
IZoneEvent | IDeviceEvent | IRouteEvent | ITripEvent | IExceptionsEvent
Event object that is sent to add-in when user interacts with different types of entitis on the map
Type TEntityEventData
IDeviceChangeEvent
Event object that is sent to add-in when something is happened with different types of entitis on the map
map service (Example)
Service for manipulating viewport of the map and getting updates about changed map viewport
Methods
Service methods | Description |
---|---|
setBounds (bounds: IMapBounds): Promise<boolean> | Sets map bounds |
getBounds (): Promise<IMapBounds> | Gets current map bounds |
setZoom (zoom: number): Promise<boolean> | Sets map zoom level |
getZoom (): Promise<number> | Gets current map zoom level |
attach (eventName: string, eventHandler: (serviceData: any) => void): void | Attaches event handler to service |
detach (eventName: string, eventHandler?: (serviceData: any) => void): void | Detaches event handler to service |
Events
Event name | Event object | Description |
---|---|---|
change | Empty object | Event is fired when viewport of map is changed. This event fires each time when use drags or zooms map |
changed | viewport: (IChangedViewport) - Current map zoom and bounds | Event is fired when user finished dragging and zooming map |
Interface IChangedViewport
Current map viewport
Property | Type | Description |
---|---|---|
zoom | number | Current map zoom |
bounds | IMapBounds | Current map bounds |
Interface IMapBounds
Object that represents a map bounding box.
Property | Type | Description |
---|---|---|
sw | IMapLatLng | The southwest corner of the bounding box. |
ne | IMapLatLng | The northeast corner of the bounding box. |
Interface IMapLatLng
An object that represents longitude and latitude
Property | Type | Description |
---|---|---|
lat | number | Latitude, measured in degrees. |
lng | number | Longitude, measured in degrees. |
tooltip service (Example)
Service for showing additional information in entities tooltip
Methods
Service methods | Description |
---|---|
showAt (position: TPosition, pattern: ITooltip, sequence: number): void | Shows custom tooltip at certain position on the map |
show (pattern: ITooltip, sequence: number): void | Adds additional information to already shown tooltip on the map |
hide (): void | Hids additional information from already shown and custom tooltip |
setConfig (config: ITooltipConfig): Promise<boolean> | Sets configuration for changing current application tooltip |
getConfig (): Promise<ITooltipConfig> | Sets configuration for current application tooltip |
Interface ITooltip
Custom map add-in tooltip options. It can be either a text information or an image
Property | Type | Description |
---|---|---|
icon | string | Icon image for custom tooltip part. |
image | ITooltipImage | Image options that should be shown instead of text in tooltip |
main | string | Main tooltip text |
secondary | string[] | Secondary information that will be written with smaller font |
additional | string[] | Some additional tooltip information |
Interface ITooltipImage
Custom tooltip image options. It can be either link to external image, base64 image or image stored in ArrayBuffer
Property | Type | Description |
---|---|---|
url | string | Either link to external image or serialized base64 image or stringified SVG image |
buffer | ArrayBuffer | Image stored in ArrayBuffer |
width | number | Width of the image |
height | number | Height of the image |
Interface ITooltipConfig
Application tooltip config. Based on this config application desides what parts of tooltip should be rendered
Property | Type | Description |
---|---|---|
device | IDeviceTooltipConfig | Changes information in devices tooltip |
Interface IDeviceTooltipConfig
Device tooltip config to control amount of information that is shown in tooltip.
Property | Type | Description |
---|---|---|
state | boolean | Shows/hides current device state in tooltip |
groups | boolean | Shows/hides device groups information in tooltip |
actionList service (Example)
Service for showing a custom action list instead of the existing one, or adding custom buttons to existing action lists.
Methods
Service methods | Description |
---|---|
show (position: TPosition, title: string, items: IMenuActionItem[]): void | Shows custom action menu on certain position |
attachMenu (menuName: TMenuType, handler: TMenuHandler): void | Subscribes on event when one of the MyGeotab map menus is shown to add new action button |
detachMenu (menuName: TMenuType, handler?: TMenuHandler): void | Unsubscribes on event when one of the MyGeotab map menus is shown |
attach (eventName: string, eventHandler: (serviceData: any) => void): void | Attaches event handler to service |
detach (eventName: string, eventHandler?: (serviceData: any) => void): void | Detaches event handler to service |
Events
Event name | Event object | Description |
---|---|---|
CustomEvent | data: (object) - Data from `IAddinActionItem.data` for the current button | Event is fired when user clicks on custom button in action list. Event name is defined by "clickEvent" property in custom button object. |
Interface IMenuActionItem
Custom action button options
Property | Type | Description |
---|---|---|
title | string | Button title |
icon | string | Button icon image |
url | string | URL to external page. If this property is used than button will be an anchor element |
clickEvent | string | Name of the event that will be fired when user clicks on this button |
zIndex | number | Number that defined position of the custom action button in menu |
data | object | Custom data that will be send with `clickEvent` when user clicks on this button |
Interface IMenuEventData
Data that is passed to add-in when all types of map action menus are about to be shown
Property | Type |
---|---|
x | number |
y | number |
menuName | string |
location | ILocation |
Interface IMapMenuEventData extends IMenuEventData
Data that is passed to add-in when map action menu is about to be shown
Interface IZoneMenuEventData extends IMenuEventData
Data that is passed to add-in when zone action menu is about to be shown
Property | Type |
---|---|
zone | { id: string; } |
Interface IRouteMenuEventData extends IMenuEventData
Data that is passed to add-in when route action menu is about to be shown
Property | Type |
---|---|
route | { id: string; } |
Interface IMarkerMenuEventData extends IMenuEventData
Data that is passed to add-in when location marker action menu is about to be shown
Property | Type |
---|---|
title | string |
Interface IDeviceMenuEventData extends IMenuEventData
Data that is passed to add-in when device action menu is about to be shown
Property | Type |
---|---|
device | { id: string; } |
Interface ITripMenuEventData extends IMenuEventData
Data that is passed to add-in when trip action menu is about to be shown
Property | Type |
---|---|
dateTime | string |
device | { id: string; } |
trip | ITripData |
Interface ITripData
Trip data that is passed to add-in when trip action menu is about to be shown
Property | Type |
---|---|
start | string |
stop | string |
Type TMenuType
"zone" | "map" | "device" | "route" | "marker" | "trip"
Types of menus where custom action buttons can be added
Type TMenuHandler
(menuName: string, data: TMenuEventData) => IMenuActionItem[] | Promise<IMenuActionItem[]>
Function that will be called when certain action menu is about to be opened
Type TMenuEventData
IMapMenuEventData | IZoneMenuEventData | IRouteMenuEventData | IMarkerMenuEventData | IDeviceMenuEventData | ITripMenuEventData
Data that is passed to add-in based on type of menu that is shown
canvas service (Example)
Service for drawing custom shapes on the map
Methods
Service methods | Description |
---|---|
path (path: IPathSeg[], zIndex: number): ICanvasElement<ICanvasPathAttributes> | Draws SVG path element on the map |
rect (coords: TPosition, width: number, height: number, radius: number, zIndex: number): ICanvasElement<ICanvasRectAttributes> | Draws SVG rect element on the map |
circle (coords: TPosition, radius: number, zIndex: number): ICanvasElement<ICanvasCircleAttributes> | Draws SVG circle element on the map |
text (coords: TPosition, text: string, zIndex: number): ICanvasElement<ICanvasTextAttributes> | Draws SVG text element on the map |
marker (coords: TPosition, width: number, height: number, source: TMarkerSource, zIndex: number): ICanvasElement<ICanvasMarkerAttributes> | Draws custom image element on the map |
clear (): void | Clears all drawn elements on the map |
Interface IPathSeg
Segment of the path element that will be added in `d` attribute
Property | Type | Description |
---|---|---|
type | string | Type of the segment. Supported types `M`, `m`, `L`, `l`, `Z`, `C`, `c`, `S`, `s` |
points | TPathSegPoint[] | Locations or coordinates that should be used in current segment |
Interface ICanvasElement<T extends TCanvasElementAttributes>
New map element object
Method | Description |
---|---|
change (attrs: T): ICanvasElement<T> | Changes style attributes of the current map element |
remove (): void | Removes current map element |
isRemoved (): boolean | Returns true if element was removed |
attach (event: TCanvasElementEvent, handler: (data: ICoordinate) => void): ICanvasElement<T> | Attaches event handler to current element event |
detach (event: TCanvasElementEvent, handler?: (data: ICoordinate) => void): ICanvasElement<T> | Detaches event handler from current element event |
Interface ICanvasElementStyleAttributes
Style properties that can be changed for every custom element
Property | Type | Description |
---|---|---|
fill | string | Background color of the element |
stroke | string | Border color of the element |
stroke-width | number | Border width of the element |
fill-opacity | number | Opacity of the element |
font-size | number | Text element font size |
font-weight | number | Text element font weight |
Interface ICanvasRectAttributes extends ICanvasElementStyleAttributes
Attribute of rect that can be changed for every custom element
Property | Type | Description |
---|---|---|
height | number | Height in pixels of the element |
width | number | Width in pixels of the element |
rx | number | Radius in pixels x-axios of the element |
ry | number | Radius in pixels y-axios of the element |
coords | TPosition | Position of the element |
Interface ICanvasTextAttributes extends ICanvasElementStyleAttributes
Text element attributes that can be changed for every custom text element
Property | Type | Description |
---|---|---|
dx | number | Offset in pixels x-axios of the element |
dy | number | Offset in pixels y-axios of the element |
text | string | Text of the element |
coords | TPosition | Position of the element |
Interface ICanvasCircleAttributes extends ICanvasElementStyleAttributes
Attribute of circle that can be changed for every custom element
Property | Type | Description |
---|---|---|
r | number | Radius in pixels of the element |
coords | TPosition | Position of the element |
Interface ICanvasPathAttributes extends ICanvasElementStyleAttributes
Attribute of path that can be changed for every custom element
Property | Type | Description |
---|---|---|
path | IPathSeg[] | Path of the element |
Interface ICanvasMarkerAttributes extends ICanvasElementStyleAttributes
Attribute of marker that can be changed for every custom element
Property | Type | Description |
---|---|---|
height | number | Height in pixels of the element |
width | number | Width in pixels of the element |
x | number | Position of the element |
y | number | Position of the element |
dx | number | Offset in pixels x-axios of the element |
dy | number | Offset in pixels y-axios of the element |
coords | TPosition | Position of the element |
href | string | Image href of the element |
buffer | ArrayBuffer | Image ArrayBuffer of the element |
Type TCanvasElementAttributes
ICanvasRectAttributes | ICanvasTextAttributes | ICanvasCircleAttributes | ICanvasPathAttributes | ICanvasMarkerAttributes
Type TCanvasElementEvent
"click" | "over" | "out"
Supported custom map element event types
Type TMarkerSource
string | ArrayBuffer
Marker can be presented as a encoded SVG or base64 string or URL to third party resource or binary array in ArrayBuffer
Interface ILocation
Property | Type |
---|---|
lat | number |
lng | number |
Interface ICoordinate
Property | Type |
---|---|
x | number |
y | number |
Type TPosition
ILocation | ICoordinate
Example Add-ins
Here are some examples and type definition files that can help you understand how to work with Map Add-ins. Download them, unzip the files, then follow the instructions in the ReadMe document.